How to Do Android App Programming: A Comprehensive Guide
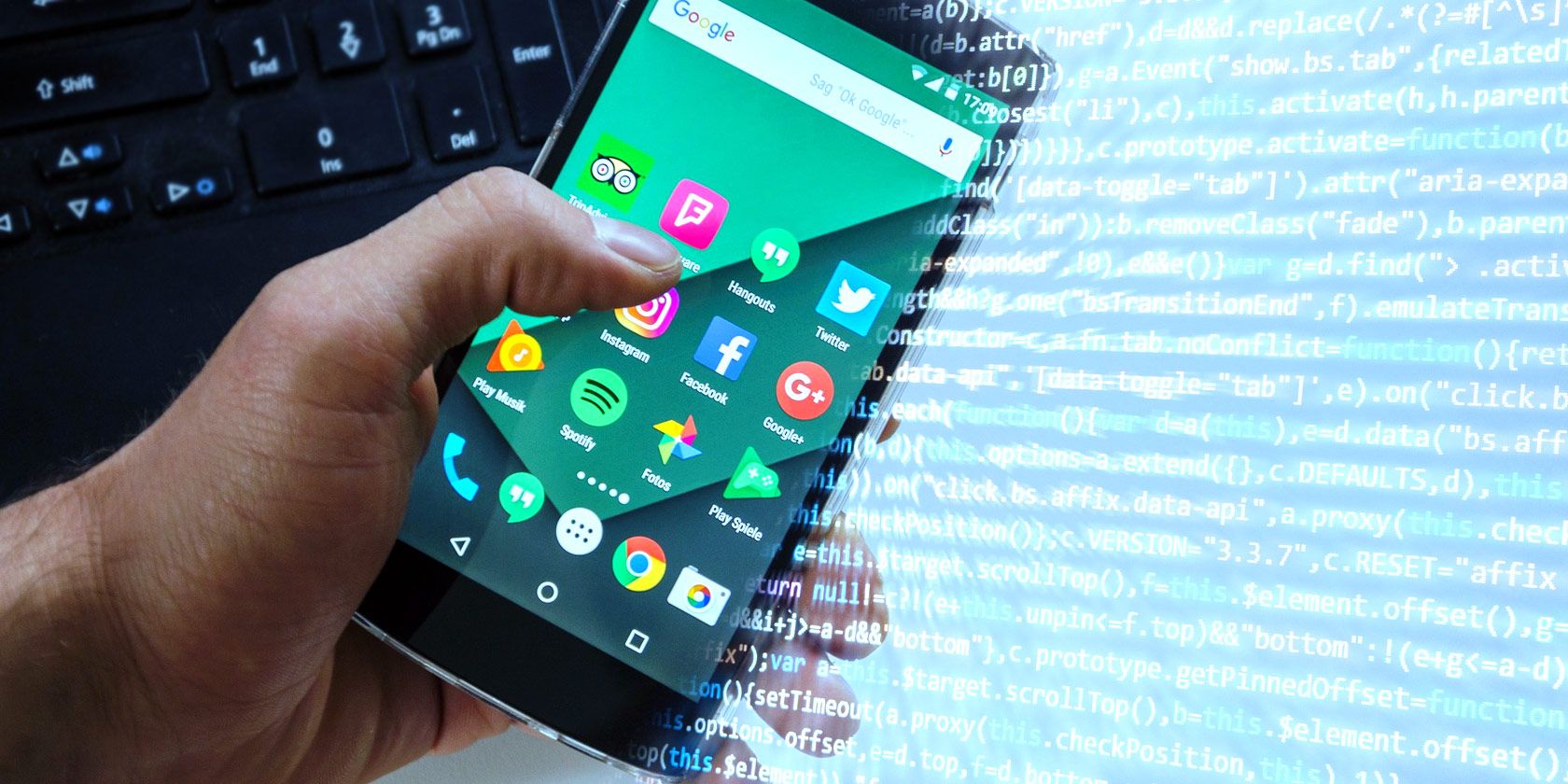
Are you eager to dive into the world of Android app programming? With over 2.5 billion active Android devices worldwide, developing apps for this platform can open up a whole new realm of opportunities for you. Whether you're a beginner or already have some programming experience, this comprehensive guide will walk you through the process of creating your very own Android app from scratch.
In this article, we will cover everything you need to know to get started with Android app programming. We'll begin by explaining the basics of Android app development, including the necessary tools and technologies. Then, we'll guide you through setting up your development environment and introduce you to the fundamentals of Java programming, which is the primary language used for Android app development.
Understanding the Android Platform
Before diving into Android app programming, it's important to have a solid understanding of the Android platform. This section will provide you with an overview of the Android ecosystem, its architecture, and the various versions available.
The Android Ecosystem
The Android ecosystem comprises the Android operating system, the Android Software Development Kit (SDK), and a wide range of devices that run on Android. The operating system is based on the Linux kernel and is designed primarily for touchscreen mobile devices such as smartphones and tablets. However, Android has also expanded to other form factors, including smartwatches, smart TVs, and even cars.
Google, the creator of Android, provides developers with the Android SDK, which includes the necessary tools and libraries for building Android apps. The SDK also includes an emulator that allows you to test your apps on virtual Android devices without the need for physical hardware.
Android Versions and Compatibility
Android has gone through several major versions, each introducing new features and improvements. It's important to consider the Android version distribution and compatibility when developing your app to ensure maximum reach.
Currently, the latest version of Android is Android 12, but it's crucial to consider the minimum API level your app will support. The API level determines which features and capabilities your app can utilize and which devices it can run on. It's generally recommended to target a minimum API level that covers a significant portion of the market while taking advantage of the latest features available in newer API levels.
Setting Up Your Development Environment
Before you can start programming Android apps, you'll need to set up your development environment. This section will guide you through the installation of the necessary software and configuring your development environment to ensure a smooth workflow.
Installing Android Studio
Android Studio is the official Integrated Development Environment (IDE) for Android app development. It provides a comprehensive set of tools for designing, building, and debugging Android apps. To get started, visit the official Android Studio website and download the latest version for your operating system.
Once the download is complete, run the installer and follow the on-screen instructions to install Android Studio. The installer will also prompt you to install the Android SDK and other necessary components. It's recommended to install the SDK components for the latest Android version as well as a few older versions to ensure compatibility with a wider range of devices.
Configuring Android Virtual Devices (AVDs)
Android Virtual Devices (AVDs) allow you to emulate different Android devices on your computer for testing purposes. Before you can run your app on an emulator, you'll need to create an AVD that matches the specifications of the device you want to emulate.
To create an AVD, open Android Studio and navigate to the "AVD Manager" from the toolbar or the "Welcome to Android Studio" screen. Click on "Create Virtual Device" and select the device definition you want to emulate, such as a Pixel phone or Nexus tablet. Then, choose the system image for the desired Android version and configure any additional settings, such as the amount of RAM and storage.
Connecting a Physical Android Device
In addition to using emulators, you can also connect a physical Android device to your computer for testing and debugging your app. To do this, enable Developer Options on your device by going to the "Settings" app, tapping on "About phone" or "About tablet," and then tapping on the "Build number" multiple times until you see a message indicating that Developer Options have been enabled.
Once you've enabled Developer Options, connect your device to your computer using a USB cable. If prompted on your device, allow USB debugging. In Android Studio, you should see your device listed in the device toolbar. You can select it as the deployment target and run your app directly on the device.
Java Basics for Android App Development
Java is the primary programming language for Android app development. This section will introduce you to the basics of Java, including variables, data types, control structures, and object-oriented programming concepts.
Variables and Data Types
In Java, variables are used to store data values that can be manipulated and accessed throughout your program. Before using a variable, you must declare its type, such as int for integers, double for floating-point numbers, or String for text.
Java supports a wide range of data types, including primitive types such as int, double, boolean, and char, as well as reference types such as String and arrays. Understanding the different data types and their limitations is essential for efficient and error-free programming.
Control Structures
Control structures allow you to control the flow of execution in your program. Java provides various control structures, including if-else statements, loops, and switch statements.
The if-else statement allows you to execute different blocks of code based on a condition. For example, you can use an if-else statement to check if a number is positive or negative and perform different actions accordingly.
Loops, such as the for loop and while loop, allow you to repeat a block of code multiple times. This is useful when you need to perform a task iteratively, such as processing elements in an array or performing calculations until a certain condition is met.
Object-Oriented Programming Concepts
Java is an object-oriented programming (OOP) language, which means it is built around objects that represent real-world entities. Understanding the core concepts of OOP is essential for developing well-structured and maintainable Android apps.
The main concepts of OOP include classes, objects, inheritance, and polymorphism. A class is a blueprint for creating objects, while an object is an instance of a class. Inheritance allows you to create new classes based on existing ones, inheriting their properties and behaviors. Polymorphism enables objects to take on multiple forms and exhibit different behaviors based on the context.
Building User Interfaces with XML
An aesthetically pleasing and user-friendly interface is crucial for any successful app. This section will teach you how to design user interfaces using XML, a markup language that allows for the creation of interactive layouts.
Understanding XML Layouts
XML (eXtensible Markup Language) is a markup language that defines the structure and content of data. In Android app development, XML is used to define the layout of user interfaces, specifying the position, size, and appearance of UI elements.
Android provides a variety of layout types, including LinearLayout, RelativeLayout, ConstraintLayout, and more. Each layout type has its own characteristics and is suited for different scenarios. Understanding the different layout types and their properties is essential for creating well-designed user interfaces.
Creating UI Elements
UI elements, also known as views, are the building blocks of Android user interfaces. In XML, you can define UI elements such as buttons, text fields, image views, and more. Each UI element is represented by a specific XML tag and can be customized with various attributes.
For example, to create a button in XML, you would use the
Applying Styles and Themes
Styles and themes allow you to define a set of visual attributes that can be applied to multiple UI elements. This promotes consistency and simplifies the process of updating the appearance of your app.
In Android, you can define styles in XML or programmatically. Styles can specify attributes such as text color, background color, font size, and more. You can then apply these styles to individual UI elements or entire activity layouts.
Handling User Input and Events
Interactivity is a key aspect of modern apps. This section will explore how to handle user input and events in your Android app, allowing users to interact with your app and providing a seamless user experience.
Getting User Input
There are various ways to capture user input in an Android app, depending on the type of input you require. For text input, you can use EditText views, which allow users to enter text using the on-screen keyboard. You can customize the behavior of EditText views, such as restricting input to specific characters or validating input.
For selecting options from a predefined set, you can use Spinner views or RadioGroup views with RadioButton options. These views present users with a list of choices and allow them tomake a selection. You can retrieve the selected option programmatically and perform actions based on the user's choice.
If you need to capture binary choices, such as on/off or yes/no, you can use CheckBox views or Switch views. These views allow users to toggle between two states, and you can retrieve the current state to determine the user's selection.
Responding to User Interactions
When users interact with UI elements in your app, various events are triggered. These events can range from button clicks to touch gestures. It's essential to handle these events appropriately to provide a responsive and intuitive user experience.
One common event is the button click event. When a user clicks a button, an onClick event is triggered. You can define a method to handle this event and perform the desired action, such as navigating to another screen or submitting a form.
Other events, such as touch events, can provide more granular control over user interactions. For example, you can capture swipe gestures, long presses, or scroll events. By implementing event listeners and callback methods, you can customize the behavior of your app based on these interactions.
Working with Data and Storage
Most apps require some form of data storage to function effectively. This section will dive into the different options for storing and retrieving data in your Android app, enabling you to create robust data management solutions.
Using Shared Preferences
Shared Preferences provide a simple and lightweight way to store small amounts of data, such as user preferences or settings. Shared Preferences are key-value pairs that can be easily accessed throughout your app. They are ideal for storing simple data types, such as strings, integers, booleans, and floats.
To use Shared Preferences, you first need to obtain an instance of the SharedPreferences class. You can then use methods like putString(), putInt(), getBoolean(), and more to store and retrieve data. This allows you to create a personalized experience for users by remembering their preferences across app sessions.
Working with Databases
If your app requires more complex data storage and retrieval, you can utilize databases. Android provides SQLite, a lightweight and efficient relational database management system built into the platform. SQLite databases are stored as files on the device and can be accessed and manipulated using SQL (Structured Query Language) queries.
To work with databases in Android, you'll need to create a subclass of the SQLiteOpenHelper class. This subclass handles the creation and management of the database, including creating tables, inserting data, updating records, and querying data. By leveraging the power of databases, you can build apps that handle large amounts of structured data efficiently.
Utilizing Cloud Storage Services
Cloud storage services allow you to store and retrieve data from remote servers, providing scalability and accessibility for your app's data. Popular cloud storage services, such as Firebase Realtime Database and Google Cloud Storage, offer easy integration with Android apps.
Using cloud storage services, you can store data in a structured manner and synchronize it across multiple devices. This enables features such as real-time collaboration, offline data access, and seamless data backup and restore. By leveraging the power of the cloud, you can create apps with enhanced functionality and a seamless user experience.
Networking and Web Services Integration
Many apps rely on network connectivity to provide valuable features and services. This section will explore how to integrate networking capabilities into your Android app, enabling seamless communication with web services and APIs.
Working with HTTP Requests
HTTP (Hypertext Transfer Protocol) is the foundation of communication on the web. Android provides the HttpURLConnection class, which allows you to make HTTP requests to remote servers and retrieve data. You can use HTTP requests to fetch data from APIs, send form data, or perform other web-related tasks.
To work with HTTP requests in Android, you'll typically create a separate thread or use asynchronous methods to avoid blocking the main UI thread. You can then use the HttpURLConnection class to establish a connection, specify the request method (GET, POST, PUT, DELETE), set request headers, and handle the response.
Parsing JSON Data
JSON (JavaScript Object Notation) is a lightweight data interchange format commonly used in web services and APIs. When working with web services, you'll often receive data in JSON format that needs to be parsed and processed in your app.
Android provides the JSONObject and JSONArray classes to parse and manipulate JSON data. You can extract values from JSON objects, iterate through arrays, and handle nested structures. By parsing JSON data, you can extract the relevant information and display it in your app's user interface.
Ensuring Secure Data Transmission
When working with network requests and web services, it's important to ensure the security of data transmission. Android provides several mechanisms to secure data, such as using HTTPS (HTTP Secure) for encrypted communication and implementing secure authentication mechanisms.
To establish secure connections using HTTPS, you'll need to obtain an SSL certificate for your server and configure your app to trust the certificate. Android provides the TrustManager and SSLContext classes to handle secure connections. Additionally, you can implement authentication mechanisms, such as token-based authentication or OAuth, to ensure that only authorized users can access your app's resources.
Enhancing App Functionality with Libraries and APIs
The Android ecosystem offers a wide range of libraries and APIs that can significantly enhance your app's functionality. This section will introduce you to popular libraries and APIs and demonstrate how to integrate them into your project.
Working with Multimedia: MediaPlayer and ExoPlayer
If your app involves playing audio or video content, you can utilize the MediaPlayer and ExoPlayer libraries. MediaPlayer provides a simple way to play audio and video files, while ExoPlayer offers more advanced features and supports a wider range of media formats.
By integrating these libraries into your app, you can provide users with a seamless multimedia experience. You can load media files from the device's storage, stream content from the web, handle playback controls, and implement features such as looping, shuffling, and seeking.
Utilizing Location Services: Google Play Services and GPS
Location-based apps are becoming increasingly popular, and Android provides robust location services for developers. The Google Play Services library offers a high-level API for accessing location information, including the device's current location, geocoding and reverse geocoding, and geofencing.
In addition to Google Play Services, you can also utilize the device's GPS (Global Positioning System) to obtain accurate location data. The Android framework provides the LocationManager class to interact with the device's GPS hardware and retrieve location updates.
Integrating Social Media: Facebook and Twitter APIs
Social media integration can enhance the reach and engagement of your app. Popular social media platforms, such as Facebook and Twitter, provide APIs that allow you to integrate their functionalities into your app.
By integrating the Facebook API, you can enable users to log in with their Facebook accounts, share content on their timelines, and access user data. Similarly, the Twitter API allows users to log in with their Twitter accounts, tweet, and retrieve user information.
Using Google Maps: Google Maps API
Google Maps is a powerful tool for displaying maps, geocoding addresses, and providing navigation capabilities in your app. The Google Maps API allows you to integrate Google Maps into your app, enabling users to view maps, search for locations, and get directions.
With the Google Maps API, you can customize the map appearance, add markers and overlays, and handle user interactions such as panning and zooming. This enables you to create location-based apps, display points of interest, and provide turn-by-turn navigation.
Testing and Debugging Your Android App
Thorough testing and debugging are essential for ensuring the quality and reliability of your app. This section will provide you with the knowledge and tools necessary to test and debug your Android app effectively.
Unit Testing with JUnit
Unit testing is a crucial aspect of app development, as it allows you to verify the correctness of individual components or units of your app. Android supports unit testing using the JUnit framework, which provides a set of tools and assertions for writing test cases.
By writing unit tests, you can validate the behavior of specific methods or classes in isolation. This helps identify and fix bugs early in the development process, ensuring that your app functions as intended.
UI Testing with Espresso
UI testing focuses on validating the behavior and appearance of your app's user interface. Android provides the Espresso testing framework, which allows you to write UI tests that interact with your app's UI elements and simulate user interactions.
Using Espresso, you can write test cases that verify the correctness of UI elements, navigation flows, and user interactions. This ensures that your app's UI behaves as expected and provides a smooth user experience.
Debugging with Android Studio
Android Studio offers powerful debugging tools that can help you identify and fix issues in your app. With the debugger, you can set breakpoints in your code, inspect variables, step through code execution, and view log messages.
When encountering a bug or unexpected behavior, you can use the debugger to analyze the state of your app and identify the root cause of the issue. This speeds up the debugging process and allows for moreefficient bug resolution.
Logging and Logcat
Logging is an essential tool for understanding the behavior of your app and diagnosing issues. Android provides the Log class, which allows you to output log messages at different levels of severity, such as verbose, debug, info, warning, and error.
By strategically placing log statements throughout your code, you can track the flow of execution, monitor variable values, and identify potential problems. The Logcat tool in Android Studio allows you to view these log messages in real-time, making it easier to troubleshoot and debug your app.
Publishing Your Android App
Once you've developed and tested your app, it's time to share it with the world. This section will guide you through the process of publishing your Android app on the Google Play Store, making it available to millions of users.
Preparing Assets and Resources
Before publishing your app, you need to ensure that all necessary assets and resources are properly prepared. This includes creating high-quality app icons, screenshots, and promotional graphics that comply with the Google Play Store's guidelines.
It's important to provide accurate and compelling descriptions of your app's features and functionality. Clear and concise text will help potential users understand what your app offers and why they should download it.
Creating a Developer Account
To publish your app on the Google Play Store, you need to create a developer account. This involves registering as a developer, agreeing to the terms and conditions, and paying a one-time registration fee.
Once your developer account is set up, you can access the Google Play Console, a web-based platform that allows you to manage and publish your app. The console provides a variety of tools and resources to help you distribute, monetize, and analyze your app's performance.
Optimizing Your App Listing
To maximize the visibility and discoverability of your app on the Google Play Store, it's crucial to optimize your app listing. This involves selecting appropriate keywords, writing an engaging app title and description, and choosing relevant and eye-catching screenshots.
Additionally, you can utilize the store listing experiments feature in the Google Play Console to A/B test different variations of your app's listing. This allows you to gather data and insights to make informed decisions about optimizing your app's presentation.
Testing Release and Rollout
Before releasing your app to the public, it's essential to thoroughly test it in a production-like environment. The Google Play Console provides a testing track where you can distribute your app to a limited group of users, such as alpha and beta testers.
By gathering feedback from testers, you can identify any remaining issues or areas for improvement. This feedback loop allows you to refine your app before the official release, ensuring a positive user experience.
Rollout Strategies and Release Management
When it comes to releasing your app, you have several rollout strategies to choose from. The Google Play Console allows you to control the release process, including staged rollouts, where you gradually release your app to a percentage of users, and managed rollouts, where you release to specific countries or devices.
Release management involves monitoring user feedback, analyzing crash reports, and iterating on your app based on user responses. By continuously improving your app and addressing any issues that arise, you can maintain a high-quality user experience.
In conclusion, this comprehensive guide has provided you with a step-by-step roadmap for Android app programming. By mastering the fundamentals of Android development, designing user interfaces, handling user input, managing data, integrating web services, utilizing libraries, and testing your app, you'll be well-equipped to create innovative and successful Android applications. So, roll up your sleeves, unleash your creativity, and embark on an exciting journey into the world of Android app programming!